Beyond the Browser: Why JavaScript is Crucial for Web Analytics
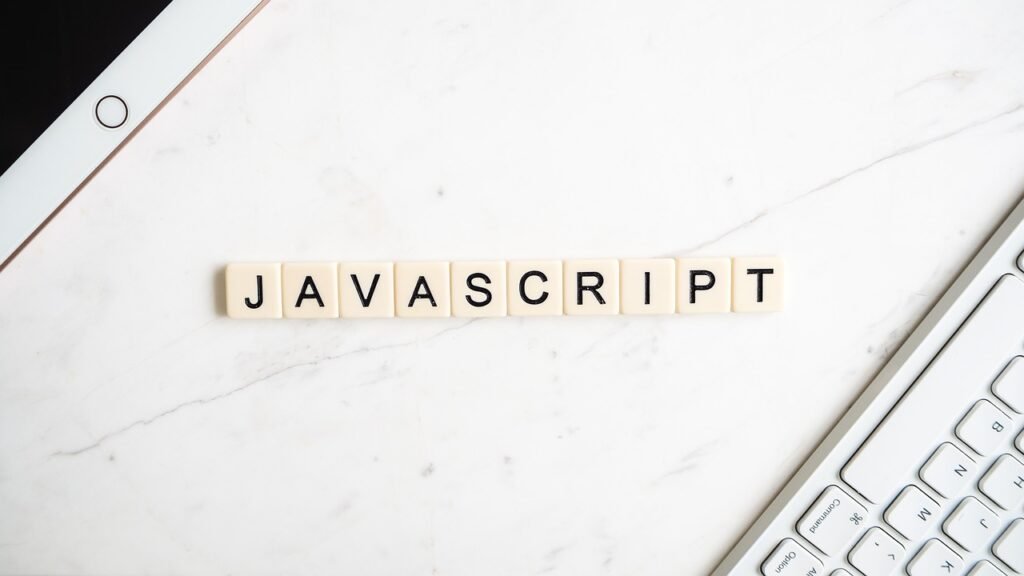
The world of web analytics is a fascinating blend of data, insights, and actionable strategies. While tools like Adobe Analytics / Google Analytics are the front-end heroes, their power relies heavily on the silent workhorse behind the scenes: JavaScript.
For the technically inclined, this blog post will delve into the critical role of JavaScript in web analytics implementation. We’ll explore how this dynamic language empowers analysts to:
1. Capture User Interactions
JavaScript allows us to track user behavior in real-time. Every click, scroll, form submission, or video playback – all these actions are meticulously captured and relayed to the analytics platform.
Example: Imagine tracking how users interact with a product page. Using JavaScript, you can set up event tracking for specific elements like “Add to Cart” buttons, product images, or even hover events. This granular data provides valuable insights into user engagement and informs optimization strategies.
// Track a click on the "Add to Cart" button
const addToCartButton = document.getElementById('add-to-cart-button');
addToCartButton.addEventListener('click', function() {
// Send event to analytics platform
ga('send', 'event', 'product', 'add_to_cart', 'product_name', {
'value': 100
});
});
2. Enrich Data with Context
JavaScript allows us to gather contextual information beyond simple page views. This includes:
- User Attributes: Data like demographics, location, and device type, enriching the user profile.
- Event Properties: Adding extra information to events. For example, tracking the specific product name when a user clicks “Add to Cart”.
- Custom Dimensions and Metrics: Defining your own metrics and dimensions tailored to your business needs.
Example: You can use JavaScript to track the time users spend on specific sections of a page or the number of times they interact with a particular element. This data can be used to identify areas of user engagement or frustration.
// Track time spent on a specific section of the page
const section = document.getElementById('product-details');
let startTime;
section.addEventListener('mouseenter', function() {
startTime = Date.now();
});
section.addEventListener('mouseleave', function() {
const endTime = Date.now();
const timeSpent = endTime - startTime;
// Send time spent to analytics platform
ga('send', 'event', 'page', 'time_on_section', 'product-details', {
'value': timeSpent
});
});
3. Customize Tracking for Specific Needs
JavaScript offers flexibility in customizing tracking solutions. This allows you to:
- Track custom events: Beyond predefined events, you can track any interaction specific to your website. This could include downloads, sign-up forms, or even interactions with a chatbot.
- Implement advanced tracking logic: Create complex tracking scenarios based on user behavior and website context.
- Integrate with other tools: JavaScript enables seamless integration with marketing automation platforms, CRM systems, and other tools, building a holistic data ecosystem.
Example: You can use JavaScript to track how many times a user views a specific ad before converting. This data can help you optimize your ad campaigns for better conversion rates.
// Track ad impressions and conversions
const adElement = document.getElementById('ad-banner');
let impressionCount = 0;
adElement.addEventListener('load', function() {
impressionCount++;
// Send impression event to analytics platform
ga('send', 'event', 'ad', 'impression', 'banner_ad');
});
// Assuming you have a conversion tracking mechanism in place
// ...
// Track conversion based on user action
if (conversionOccurred) {
// Send conversion event to analytics platform
ga('send', 'event', 'ad', 'conversion', 'banner_ad', {
'value': 10
});
}
4. Real-time Insights and Dynamic Reporting
JavaScript allows for real-time data processing and analysis. This enables:
- Dynamically updating reports: Displaying live insights based on user activity.
- Triggering personalized experiences: Tailoring website content or offers based on real-time user behavior.
- A/B Testing: Running experiments with real-time data analysis to optimize website elements.
Example: You can use JavaScript to create a dynamic pop-up that offers a discount to users who are about to abandon their shopping cart.
// Monitor user behavior for cart abandonment
const cartButton = document.getElementById('cart-button');
cartButton.addEventListener('click', function() {
setTimeout(function() {
// Check if user has interacted with checkout process
// ...
if (!userInteractedWithCheckout) {
// Display a pop-up offering a discount
// ...
}
}, 3000); // Check after 3 seconds of inactivity
});
Challenges and Best Practices
While JavaScript is incredibly powerful, it’s crucial to implement it responsibly:
- Performance optimization: JavaScript code can affect website load times. Ensure your code is optimized for speed and efficiency.
- Privacy considerations: Be mindful of user data and ensure compliance with privacy regulations like GDPR.
- Code quality and testing: Thoroughly test your JavaScript code to avoid errors and ensure accurate tracking.
Conclusion
JavaScript is the backbone of modern web analytics. Its ability to track user interactions, enrich data, and customize tracking solutions empowers businesses to gain valuable insights and drive meaningful results. By embracing JavaScript, analysts can unlock the full potential of their data and make informed decisions to improve user experience and achieve business goals.